オーバーレイの作成
ここではライブ画像上にテキストや図を描く方法について説明します。
今回のサンプルプログラムのC#用のソースコードはMy Documents/IC Imaging Control 3.5内の以下のディレクトリに格納されています。
samples\C# *\Creating an Overlay
プロジェクトの新規作成
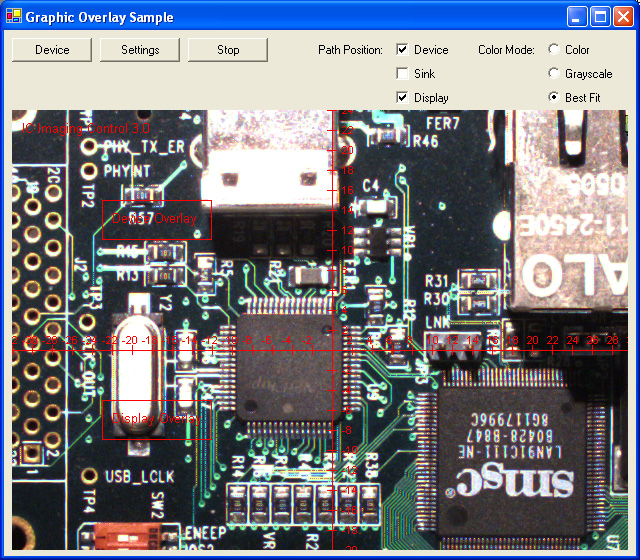
まず最初に、はじめに: Visual Studio .NETプログラマーズガイド>Visual Studioでスタートで説明してある通りにプロジェクトを新規作成して、IC Imaging Controlをフォームに追加します。
フォームに3つのボタンを追加し、CaptionプロパティをそれぞれDevice, Start, Settingsとします。
またcmdSelectDevice,cmdLiveVideo,cmdImageSettingと名前を付けます。これらのボタンは画像取り込みデバイスの選択、ライブ表示の開始と停止、そして画像設定ダイアログを表示するのに使用されます。

では3つのボタンにハンドラを実装しましょう。
private void cmdDevice_Click( object sender, EventArgs e )
{
cmdStartStop.Text = "Start";
bool wasLive = icImagingControl1.LiveVideoRunning;
if ( wasLive )
icImagingControl1.LiveStop();
icImagingControl1.ShowDeviceSettingsDialog();
if ( icImagingControl1.DeviceValid )
{
if ( wasLive )
{
icImagingControl1.LiveStart();
cmdStartStop.Text = "Stop";
}
cmdStartStop.Enabled = true ;
cmdSettings.Enabled = true ;
}
}
private void cmdStartStop_Click( object sender, EventArgs e )
{
if ( icImagingControl1.LiveVideoRunning )
{
icImagingControl1.LiveStop();
cmdStartStop.Text = "Start";
}
else
{
if( icImagingControl.DeviceValid )
{
icImagingControl1.LiveStart();
cmdStartStop.Text = "Stop";
}
}
}
private void cmdSettings_Click( object sender, EventArgs e )
{
if ( icImagingControl1.DeviceValid )
{
icImagingControl1.ShowPropertyDialog();
}
}
プログラムを実行してください。これで画像取り込みデバイスを選択し、ライブ画像が見られるようになったかと思います。
オーバーレイオブジェクトを使用する
IC Imaging Controlはライブ画像に図や文字を書き込める特殊なオブジェクトを提供しています。
それはOverlayBitmapクラスライブラリリファレンス>クラス>OverlayBitmapと呼ばれます。
OverlayBitmapクラスライブラリリファレンス>クラス>OverlayBitmapオブジェクトはbitmapを含んでおり、そのbitmapはビデオフォーマット、もしくはフレームフィルタのサイズと同になります。アプリケーションはテキストや図をこのoverlay bitmap上に描くことができ、それがビデオのの各フレームへコピーされます。ドロップアウトカラーを指定することでoverlay bitmapの特定のピクセルを透明にし、ライブ画像上で表示されないようにすることもできます。
overlay bitmapはビデオフォーマットが変更されてから少なくとも一度はICImagingControl.LiveStartクラスライブラリリファレンス>クラス>ICImagingControl>ICImagingControl.LiveStart Method
が呼び出されてからのみ使用することが可能です。ライブ表示中のみ見えるようになっています。
オーバレイの有効、無効化(デバイス、シンク、ディスプレイパス)
version 3.0以降、 IC Imaging Controlではライブ表示、または取り込み/録画用の画像上、もしくはその両方に描画するよう選択可能となっています。
ICImagingControl.OverlayBitmapPositionクラスライブラリリファレンス>クラス>ICImagingControl.OverlayBitmapPosition Property
を使ってPathPositionsクラスライブラリリファレンス>エニュメレーション(列挙)>refenum.PathPositions
の値を設定して、overlay bitmapオブジェクトをどのパスに挿入するかを指定することができます。
overlay bitmapを有効にするのには2つの処理を行います:
ライブ表示を開始する前にOverlayBitmapPositionクラスライブラリリファレンス>クラス>ICImagingControl>ICImagingControl.OverlayBitmapPosition Property
を設定し、どこのパスで使用するかを決定します。
OverlayBitmap.Enableクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.Enable Property
によってライブ表示中に特定のoverlay bitmapを有効化、無効化します。
OverlayBitmap オブジェクトにアクセスする
ICImagingControl.OverlayBitmapクラスライブラリリファレンス>クラス>ICImagingControl>ICImagingControl.OverlayBitmap Propertyプロパティはデバイスパスの OverlayBitmapクラスライブラリリファレンス>クラス>OverlayBitmap を返します。
オーバーレイのセットアップ
ICImagingControl.LivePreparedクラスライブラリリファレンス>クラス>ICImagingControl>ICImagingControl.LivePrepared Event イベントはライブモードの準備が完了した時に発生します。3つのoverlay bitmapに複数の図柄を描画するイベントハンドラを実装しましょう。
private void icImagingControl1_LivePrepared( object sender, EventArgs e )
{
if ( icImagingControl1.DeviceValid )
{
if ( (icImagingControl1.OverlayBitmapPosition & PathPositions.Device) != 0 )
{
SetupOverlay( icImagingControl1.OverlayBitmapAtPath[PathPositions.Device] );
// 座標システムを表示
DrawCoordinatesystem( icImagingControl1.OverlayBitmapAtPath[PathPositions.Device] );
// Overlay Info BOX を描画
DrawOverlayInfo( icImagingControl1.OverlayBitmapAtPath[PathPositions.Device] );
}
if( (icImagingControl1.OverlayBitmapPosition & PathPositions.Sink) != 0 )
{
SetupOverlay( icImagingControl1.OverlayBitmapAtPath[PathPositions.Sink] );
// Overlay Info BOX を描画
DrawOverlayInfo( icImagingControl1.OverlayBitmapAtPath[PathPositions.Sink] );
}
if( (icImagingControl1.OverlayBitmapPosition & PathPositions.Display) != 0 )
{
SetupOverlay( icImagingControl1.OverlayBitmapAtPath[PathPositions.Display] );
// ビットマップファイルをロードしディスプレイオーバーレイに表示
ShowBitmap( icImagingControl1.OverlayBitmapAtPath[PathPositions.Display] );
// Overlay Info BOX を描画
DrawOverlayInfo( icImagingControl1.OverlayBitmapAtPath[PathPositions.Display] );
}
}
}
SetupOverlayサブ関数はライブモードが開始された後にコールされ、3つのオーバーレイを初期化します。
private void SetupOverlay( TIS.Imaging.OverlayBitmap ob )
{
// overlay bitmap を有効化し描画可能にする
ob.Enable = true;
// Magenta(マゼンタ)を ドロップアウトカラーとする
ob.DropOutColor = Color.Magenta;
// overlay bitmap をドロップアウトカラーで塗りつぶす
ob.Fill( ob.DropOutColor );
// テキストを赤色で描く
ob.FontTransparent = true ;
ob.DrawText( Color.Red, 10, 10, "IC Imaging Control 3.x" );
}
オーバーレイはOverlayBitmap.Enableクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.Enable Propertyを呼び出すことで有効になり、テキストがbitmapの左上の隅に表示されます。サブ関数はドロップアウトカラーも設定しています。
ドロップアウトカラー
OverlayBitmap オブジェクトのOverlayBitmap.DropOutColorクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DropOutColor Propertyプロパティはライブ画像上のどの色を透明にするかを決めます。ドロップアウトカラーを持つピクセルは全てライブ画像にコピーされなくなります。ドロップアウトカラーは Visual BasicのRGB関数で設定できます。デフォルトでは黒に設定されているため、上にあるようにテキストの背景は透明になっています。OverlayBitmapオブジェクトのFontBackColorクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.FontBackColor Property プロパティもデフォルトでは黒に設定されています。
オーバーレイで黒を使えるようにするには OverlayBitmap.DropOutColorクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DropOutColor Propertyを別の色に設定します。例としてマゼンタにするとします(RGB(255,0,255))。アプリケーションを開始すると黒い画面とテキストのみが表示されるようになります。画面が黒くなるのはoverlay bitmapの色が黒でドロップアウトカラーはマゼンタに変更されたからです。よって背景を透明にするためにはoverlay bitmapの色をマゼンタで塗りつぶさなければなりません。OverlayBitmap.Fillクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.Fill Method メソッドがこの機能を果たします。ドロップアウトカラーが変更されると、テキストの背景は黒い長方形として見えるようになります。これを見えないようにするためには、プロパティOverlayBitmap.FontTransparentクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.FontTransparent Property をTrueに設定します。
座標面を描く
次のステップでは線とラベルで構成される座標面を描きます。これにはOverlayBitmapオブジェクトにあるDrawLineクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DrawLine Method と DrawTextクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DrawText Method メソッドを使用します。また、ラベルに使用する文字のフォントの変更も行いたいと思います。
では新しいサブ関数、DrawCoordinatesystemを実装しましょう。ラベルのフォントには8dpiのArialを使用したいので、 新しいStdFontオブジェクトであるFontを作成しなければなりません。このオブジェクトは名前、サイズ、他の属性を決定するフォントプロパティを持っています。
フォントを変更する前に現在のフォント設定を保存しておき、座標面が完成した後に復元できるようにしておきましょう。
OverlayBitmapオブジェクトのFontクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.Font PropertyプロパティはStdFont オブジェクトを受け取ります。これで新しいフォントが以下のOverlayBitmap.DrawTextクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DrawText Methodの呼び出しの際に使えます。
完成した座標面を描くサブ関数は以下の通り実装されます。
private void DrawCoordinatesystem( TIS.Imaging.OverlayBitmap ob )
{
// 画像の中心を算出する
int Col = GetVideoDimension().Width / 2;
int Row = GetVideoDimension().Height / 2;
Font OldFont = ob.Font;
ob.Font = new Font( "Arial", 8 );
ob.DrawLine( Color.Red, Col, 0, Col, GetVideoDimension().Height );
ob.DrawLine( Color.Red, 0, Row, GetVideoDimension().Width, Row );
for ( int i = 0; i < Row; i += 20 )
{
ob.DrawLine( Color.Red, Col - 5, Row - i, Col + 5, Row - i );
ob.DrawLine( Color.Red, Col - 5, Row + i, Col + 5, Row + i );
if ( i > 0 )
{
ob.DrawText( Color.Red, Col + 10, Row - i - 7, string.Format( "{0}", i / 10 ) );
ob.DrawText( Color.Red, Col + 10, Row + i - 7, string.Format( "{0}", -i / 10 ) );
}
}
for ( int i = 0; i < Col; i += 20 )
{
ob.DrawLine( Color.Red, Col - i, Row - 5, Col - i, Row + 5 );
ob.DrawLine( Color.Red, Col + i, Row - 5, Col + i, Row + 5 );
if ( i > 0 )
{
ob.DrawText( Color.Red, Col + i - 5, Row - 17, string.Format( "{0}", i / 10 ) );
ob.DrawText( Color.Red, Col - i - 10, Row - 17, string.Format( "{0}", -i / 10 ) );
}
}
ob.Font = OldFont;
}
現在の時刻を表示させる
private void timer1_Tick( object sender, EventArgs e )
{
TIS.Imaging.OverlayBitmap ob = icImagingControl1.OverlayBitmapAtPath[TIS.Imaging.PathPositions.Device];
if ( icImagingControl1.LiveVideoRunning )
{
// 現在のフォントを保存
Font OldFont = ob.Font;
ob.Font = new Font( "Arial", 14, FontStyle.Bold );
// 画像の左下に時間を表示させる
int Col = GetVideoDimension().Width - 81;
int Row = GetVideoDimensin().Height - 20;
// 背景の色と描画モードをセット
ob.FontTransparent = false;
ob.FontBackColor = Color.Black;
// 現在の時刻を白色で表示させる
ob.DrawText( Color.White, Col, Row, DateTime.Now.ToString( "T" ) );
// 前に使用していたフォントの呼び出し
ob.Font = OldFont;
}
}
OverlayUpdate イベント
IC Imaging ControlではOverlayUpdaterクラスライブラリリファレンス>クラス>OverlayBitmap>ICImagingControl.OverlayUpdate Eventイベントを用意しています。このイベントは画像取り込みデバイスより新しいフレームが転送された後に呼び出されます。よってフレームカウンターのようにフレームごとに違う情報を挿入することが可能となります。
例にあるOverlayUpdateクラスライブラリリファレンス>クラス>OverlayBitmap>ICImagingControl.OverlayUpdate Event サブ関数は変数FrameCountでフレーム数をカウントします。これは三角形のゲージを描くのに使用されます。もしカウンターが25を超えた場合、0にリセットされ三角形が消去されます。よって同じサイズで単一色の長方形をその三角形の上から描画する場合は、その長方形の色はDropOutColorクラスライブラリリファレンス>クラス>OverlayBitmap>ICImagingControl.OverlayUpdate Eventとして設定し、透過させなければなりません。DrawSolidRectクラスライブラリリファレンス>クラス>OverlayBitmap>OverlayBitmap.DrawSolidRect Method メソッドがこの機能を提供します。
OverlayUpdateクラスライブラリリファレンス>クラス>OverlayBitmap>ICImagingControl.OverlayUpdate Eventイベントハンドラは次の通り実装されます。
private void icImagingControl1_OverlayUpdate( object sender, TIS.Imaging.ICImagingControl.OverlayUpdateEventArgs e )
{
TIS.Imaging.OverlayBitmap ob = e.overlay;
var info = icImagingControl1.DriverFrameDropInformation;
int frameCount = (int)info.FramesDelivered;
int lineIndex = frameCount % 25;
if ( lineIndex == 0 )
{
ob.DrawSolidRect( ob.DropOutColor, 10, GetVideoDimension().Height - 70, 62, GetVideoDimension().Height - 9 );
}
ob.DrawLine( Color.Yellow, lineIndex * 2 + 10, GetVideoDimension().Height - 10, lineIndex * 2 + 10, GetVideoDimension().Height - 10 - lineIndex );
// 現在のフレームナンバーを表示
// 背景色をドロップアウトカラーに設定し、フォントは非透明にする
ob.FontBackColor = ob.DropOutColor;
ob.FontTransparent = false;
// 現在のフォント設定を保存する
Font OldFont = ob.Font;
// テキストを描く
ob.Font = new Font( "Arial", 8 );
ob.DrawText( Color.Yellow, 70, GetVideoDimension().Height - 19, frameCount.ToString() );
// フォントを元に戻す
ob.Font = OldFont;
}
C#のGraphics関数
overlay bitmapにビットマップ画像を描画するための新しいサブ関数ShowBitmapを実装します。OverlayBitmap.GetGraphicsクラスライブラリリファレンス>クラス>OverlayBitmap.GetGraphics Methodメソッドを使ってoverlay bitmapを描画するための.NET Graphicsオブジェクトを呼び出します。
private void ShowBitmap( TIS.Imaging.OverlayBitmap ob )
{
try
{
// サンプルのビットマップをプロジェクトファイルのディレクトリからロードする
var bmp = Creating_an_Overlay.Properties.Resources.hardware;
// 縦の列を計算してビットマップをウィンドウの右上に表示する
int col = GetVideoDimension().Width - 5 - bmp.Width;
// OverlayBitmapのGraphicsオブジェクトを呼び出す
Graphics g = ob.GetGraphics();
// 画像を描画する
g.DrawImage( bmp, col, 5 );
// 描画が完了したら、Graphicsオブジェクトを開放する
ob.ReleaseGraphics( g );
}
catch( Exception Ex )
{
MessageBox.Show( "File not found: " + Ex.Message );
}
}
オーバーレイのパスの位置を設定する
このサンプルでは3つのoverlay bitmapのうちどこにオーバーレイを描画するかをコントロールすることができます。パスの位置クラスライブラリリファレンス>エニュメレーション(列挙)>refenum.PathPositionsを変更をするために、以下のヘルパーサブ関数を使います。
private void EnableOverlayBitmapAtPath( PathPositions pos, bool enabled )
{
bool wasLive = icImagingControl1.LiveVideoRunning;
if( wasLive )
icImagingControl1.LiveStop();
PathPositions oldPos = icImagingControl1.OverlayBitmapPosition;
if ( enabled )
icImagingControl1.OverlayBitmapPosition = oldPos | pos;
else
icImagingControl1.OverlayBitmapPosition = oldPos & ~pos;
if ( wasLive )
icImagingControl1.LiveStart();
}
このサブにより、特定のパスポジションにオーバーレイを有効、または無効にします。
オーバーレイのカラーモードを設定する
アプリケーションはオーバーレイをグレースケールかカラーのどちらで表示するかを選択できます。OverlayBitmap.ColorModeクラスライブラリリファレンス>クラス>OverlayBitmap.ColorMode Propertyプロパティを使って下のいずれかのカラーモードを選択できます。
■ OverlayBitmap.OverlayColorModes.BestFit
overlay bitmapフォーマットは画像ストリームに合わせて最適なものが選択されます。
■ OverlayBitmap.OverlayColorModes.Color
カラーのoverlay bitmap フォーマットです。
オーバレイにカラーの線やテキストを描くことができます。
■ OverlayBitmap.OverlayColorModes.Grayscale
グレースケールのoverlay bitmap フォーマットです。
線やテキストはグレースケールでしか描けません。
画像データストリームがoverlay bitmap カラーモードに対応しない時には、その画像データは自動的に変換されます。